Start Using JavaScript
JavaScript Introduction:
1. What is JavaScript ?
- JavaScript is world's most popular scripting or programming language.
- JavaScript is the language of web.
- JavaScript is easy to learn.
- It makes the web pages more interactive.
- Short name of JavaScript is js.
2. Why Study JavaScript ?
- JavaScript is one of the 3 languages that all web developers must learn.
- We can make our Webpages more alive by manipulating HTML DOM(Document Object Model).
- JavaScript can add/change/remove HTML elements.
- JavaScript can add/change/remove HTML attributes.
- JavaScript can add/change/remove CSS styles.
- JavaScript can react to HTML events.
- JavaScript can add/change/remove HTML events.
3. What is JavaScript used for ?
- Adding interactive behavior to web pages.
- Creating web and mobile apps.
- Building web servers and developing server applications.
- Game development.
4. SO, What is a computer Program ?
=> A computer program is a list of "instructions" to be "executed" by a computer. In a programming language, these programming instructions are called statements. A JavaScript program is a list of programming statements.
Point To Remember: In HTML, JavaScript programs are executed by the web browser.
The HTML DOM (Document Object Model):-
When a web page is loaded, the browser creates a Document Object Model of the page.
The HTML DOM model is constructed as a tree of Objects:
The HTML DOM Tree of Objects
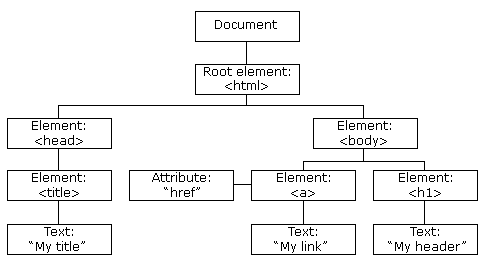
How to use JavaScript in our web pages ?
You can use javascript in your HTML DOCUMENT as shown in the following example:-
Example:
Use <script>//JavaScript Codes goes here.....</script> tag to write your javascript code.
<html>
<head><title>Webpage</title>
<body>
<h1>This is a JavaScript Example </h1>
<script>alert("Hello World");</script>
</body>
</html>
Output of above code:
Note: You can use <script> tag inside of <head> tag or inside of <body> tag .
This is an Example of Internal JavaScript.
External JavaScript:-
Just like CSS we can write our javascript code in another file and link it in our HTML documents. The file's extension should be "fileName.js".
After creating javascript file we have to link our javascript file to our HTML file as shown in the
example below.
Example:-
fileName.js
<!DOCTYPE html>
<html>
<head>
<title>Web Page</title>
<body>
<h2>External JavaScript Example</h2>
<p>This is a parageaph </p>
<script src="fileName.js"></script>
</body>
</html>
We Have Seen "alert()" function in JavaScript. Lets explore some more functions in JavaScript:
First of let me tell you that we will be using the "alert()" function to print the result in just some cases otherwise we won't be using "alert()" function that often. So, What functions we will be using?
We will be using "console.log()" function to print the result.
Example of "console.log()":
<!DOCTYPE html>
<html>
<head>
<title>Web Page</title>
<body>
<h2>External JavaScript Example</h2>
<p>This is a parageaph </p>
<script >console.log("I am printed through console.log function "</script>
</body>
</html>
Save the file. Now open it in chrome or Firefox browser and follow the following steps:
- Right Click on the HTML DOM and click "Inspect" Or press "Ctrl + Shift + J (Windows/Linux) & Command + Option + J (Mac)" in Chrome and press in Firefox "Ctrl + Shift + J (Windows/Linux) & Command + Shift + J (Mac)". Search for "Console" in the new window at right side of your chorme. Have a look at the screenshot below:
ScreenShot:-

Output of the code should be:-
Manipulating HTML DOM using JavaScript:-
If you want to manipulate HTML DOM with javascript then first you have to access HTML Elements.
There are a couple of ways to do this:-
- Finding HTML elements by id
- Finding HTML elements by tag name
- Finding HTML elements by class name
- Finding HTML elements by CSS selectors
- Finding HTML elements by HTML object collections
Finding HTML Element by Id:-
<!DOCTYPE html>
<html>
<head>
<title>Web Page</title>
<body>
<h2 id="myhtmlelement"></h2>
<script >document.getElementById("Heading 2")</script>
</body>
</html>
Comments
Post a Comment